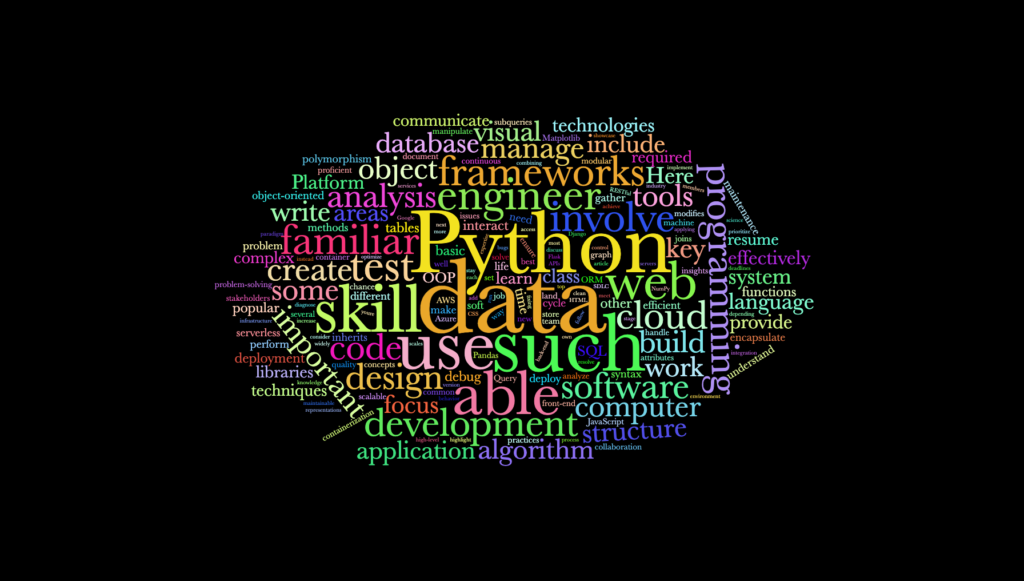
Master Your Python Interview with These Essential Q&A Tips
Python continues to dominate the tech industry, powering applications in fields ranging from web development to machine learning. Its simplicity and versatility make it a favorite among developers and employers alike. For candidates preparing for Python interviews, understanding the commonly asked questions and how to answer them effectively can be the key to landing your dream job.
This guide covers essential Python interview questions and answers, categorized for beginners, intermediates, and experts. Let’s dive in!
1. Basic Python Interview Questions
Q1: What is Python, and what are its key features?
Answer: Python is a high-level, interpreted programming language known for its readability and simplicity. Key features include:
- Easy syntax, similar to English.
- Dynamically typed (no need to declare variable types).
- Extensive standard libraries.
- Cross-platform compatibility.
- Supports multiple paradigms: object-oriented, procedural, and functional.
Q2: What are Python’s data types?
Answer: Python offers the following built-in data types:
- Numeric: int, float, complex
- Sequence: list, tuple, range
- Text: str
- Set: set, frozenset
- Mapping: dict
- Boolean: bool
- Binary: bytes, bytearray, memoryview
Q3: Explain Python’s Global Interpreter Lock (GIL).
Answer: The Global Interpreter Lock (GIL) is a mutex that protects access to Python objects, preventing multiple native threads from executing Python bytecode simultaneously. This ensures thread safety but can limit multithreading performance in CPU-bound tasks.
Q4: What are Python’s popular frameworks?
Answer: Some popular Python frameworks include:
- Web Development: Django, Flask, FastAPI
- Data Science: TensorFlow, PyTorch, Pandas
- Automation: Selenium, Robot Framework
2. Intermediate Python Interview Questions
Q5: What is the difference between shallow and deep copying?
Answer:
- Shallow Copy: Creates a new object but inserts references to the original objects within it. Use
copy.copy()
. - Deep Copy: Creates a new object and recursively copies all objects within it. Use
copy.deepcopy()
.
Q6: What are Python decorators?
Answer: Decorators are functions that modify the behavior of another function or method. They are applied using the @decorator_name
syntax and are commonly used for:
- Logging
- Authentication
- Performance measurement
- Access control
Example:
def decorator(func):
def wrapper():
print("Before function execution")
func()
print("After function execution")
return wrapper
@decorator
def say_hello():
print("Hello!")
say_hello()
Q7: How is memory managed in Python?
Answer: Python uses automatic memory management through:
- Reference Counting: Tracks the number of references to an object.
- Garbage Collection: Reclaims memory when objects are no longer in use.
- Memory Pools: Allocates memory blocks to improve efficiency.
3. Advanced Python Interview Questions
Q8: Explain Python’s metaclasses.
Answer: Metaclasses define how classes behave. They control class creation and are specified using the metaclass
keyword in class definitions. Metaclasses are commonly used to:
- Enforce coding standards.
- Add methods or attributes dynamically.
- Perform validation during class creation.
Q9: What are Python’s comprehensions?
Answer: Comprehensions provide a concise way to create sequences. Types include:
- List Comprehension:
[x for x in range(10)]
- Set Comprehension:
{x for x in range(10)}
- Dictionary Comprehension:
{x: x**2 for x in range(10)}
- Generator Expression:
(x for x in range(10))
Q10: How can you optimize Python code?
Answer:
- Use built-in functions and libraries.
- Apply list comprehensions instead of loops.
- Use generators for large datasets.
- Leverage caching with
functools.lru_cache
. - Profile code using
cProfile
and optimize hotspots.
Python Coding Challenges for Interviews
Challenge 1: Reverse a String
Write a function to reverse a string without using built-in functions.
def reverse_string(s):
result = ""
for char in s:
result = char + result
return result
Challenge 2: FizzBuzz Problem
Print numbers from 1 to 100. For multiples of 3, print “Fizz”; for multiples of 5, print “Buzz”; for multiples of both, print “FizzBuzz”.
for i in range(1, 101):
if i % 3 == 0 and i % 5 == 0:
print("FizzBuzz")
elif i % 3 == 0:
print("Fizz")
elif i % 5 == 0:
print("Buzz")
else:
print(i)
Challenge 3: Find Duplicates in a List
Write a function to find duplicate elements in a list.
def find_duplicates(lst):
seen = set()
duplicates = set()
for item in lst:
if item in seen:
duplicates.add(item)
else:
seen.add(item)
return list(duplicates)
Conclusion
Preparing for a Python interview requires a mix of theoretical knowledge and hands-on practice. The questions above cover a wide range of topics, ensuring you are well-equipped for technical discussions. Remember, employers value problem-solving skills and clear communication as much as technical proficiency. Practice consistently, and you’ll be ready to ace that Python interview!