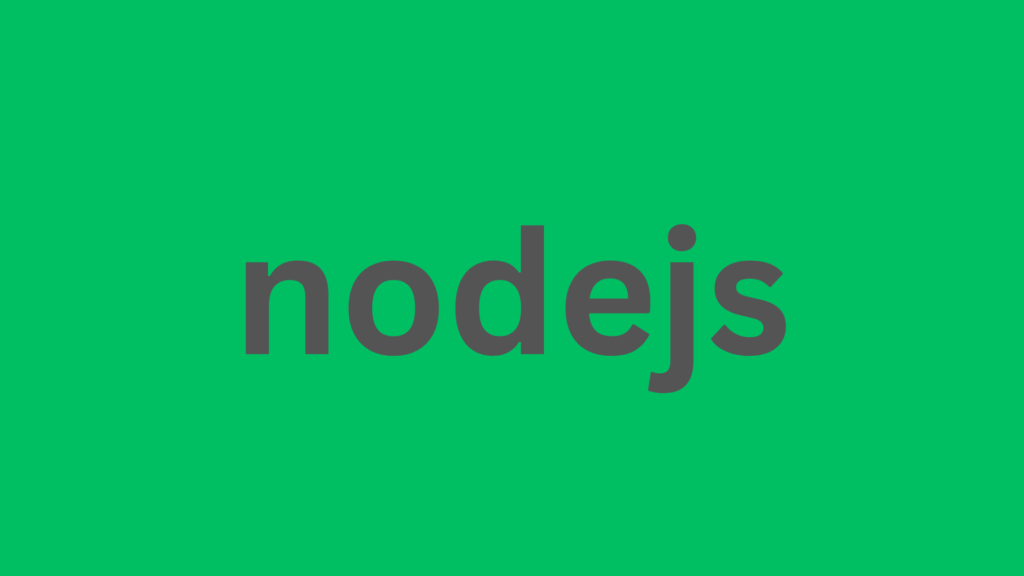
Master Your Next Node.js Interview: Expert Tips and Answers!
In today’s tech-driven world, Node.js has emerged as one of the most sought-after technologies for backend development. If you’re preparing for a Node.js interview, having a clear understanding of key concepts, as well as the ability to answer challenging questions, can make all the difference.
This guide covers a mix of basic, intermediate, and advanced Node.js interview questions to help you ace your next interview.
1. What is Node.js, and Why is it Popular?
Node.js is a runtime environment built on Chrome’s V8 JavaScript engine. It allows developers to use JavaScript on the server side, enabling full-stack development with a single programming language.
Key Reasons for Popularity
- Event-Driven Architecture: Efficiently handles asynchronous operations.
- Scalability: Ideal for building scalable network applications.
- Large Ecosystem: NPM offers access to thousands of packages.
- Cross-Platform: Compatible with Windows, macOS, and Linux.
2. Explain the Event Loop in Node.js.
The event loop is the mechanism that allows Node.js to perform non-blocking I/O operations.
How It Works:
- The event loop continuously checks the event queue for tasks.
- It delegates I/O operations to the system and processes callbacks once the operations are complete.
Example:
javascriptCopy codeconsole.log('Start');
setTimeout(() => console.log('Async Task'), 1000);
console.log('End');
// Output: Start, End, Async Task
3. What are Streams in Node.js?
Streams are objects that facilitate reading and writing data efficiently.
Types of Streams:
- Readable Streams: Data can be read from them (e.g.,
fs.createReadStream
). - Writable Streams: Data can be written to them (e.g.,
fs.createWriteStream
). - Duplex Streams: Both readable and writable (e.g., network sockets).
- Transform Streams: Modify or transform the data (e.g.,
zlib.createGzip
).
Code Example:
javascriptCopy codeconst fs = require('fs');
const readStream = fs.createReadStream('input.txt');
const writeStream = fs.createWriteStream('output.txt');
readStream.pipe(writeStream);
4. What is Middleware in Express.js?
Middleware functions in Express.js execute during the request-response cycle.
Types of Middleware:
- Application-Level Middleware: Bound to an instance of
express()
. - Router-Level Middleware: Bound to an instance of
express.Router()
. - Error-Handling Middleware: Handles errors within the app.
- Built-In Middleware: Provided by Express (e.g.,
express.static
).
Example:
javascriptCopy codeapp.use((req, res, next) => {
console.log(`Request Method: ${req.method}`);
next();
});
5. How Does Node.js Handle Asynchronous Programming?
Node.js uses callbacks, Promises, and async/await
to handle asynchronous operations.
Callback Example:
javascriptCopy codefs.readFile('file.txt', (err, data) => {
if (err) throw err;
console.log(data.toString());
});
Promise Example:
javascriptCopy codefs.promises.readFile('file.txt')
.then(data => console.log(data.toString()))
.catch(err => console.error(err));
Async/Await Example:
javascriptCopy codeasync function readFile() {
try {
const data = await fs.promises.readFile('file.txt');
console.log(data.toString());
} catch (err) {
console.error(err);
}
}
6. What is the Difference Between process.nextTick()
and setImmediate()
?
process.nextTick()
: Executes tasks before the next event loop iteration.setImmediate()
: Schedules tasks to run in the next iteration of the event loop.
Example:
javascriptCopy codeprocess.nextTick(() => console.log('Next Tick'));
setImmediate(() => console.log('Immediate'));
console.log('End');
// Output: End, Next Tick, Immediate
7. What is Clustering in Node.js?
Clustering allows Node.js to utilize multiple CPU cores by creating child processes that share the same server port.
Example of Clustering:
javascriptCopy codeconst cluster = require('cluster');
const http = require('http');
if (cluster.isMaster) {
for (let i = 0; i < 4; i++) {
cluster.fork();
}
} else {
http.createServer((req, res) => {
res.writeHead(200);
res.end('Hello World');
}).listen(8000);
}
8. How Do You Secure a Node.js Application?
Best Practices for Security:
- Use HTTPS: Encrypt data in transit.
- Environment Variables: Store sensitive information securely.
- Input Validation: Prevent injection attacks.
- Rate Limiting: Prevent brute-force attacks.
- Use Helmet: Secure HTTP headers in Express.js apps.
Example:
javascriptCopy codeconst helmet = require('helmet');
app.use(helmet());
Conclusion
Mastering Node.js concepts is essential for cracking interviews and excelling as a backend developer. This guide provides a comprehensive overview of commonly asked Node.js questions, along with clear and concise answers. With adequate preparation and a solid understanding of these topics, you’ll be ready to tackle any Node.js interview with confidence.