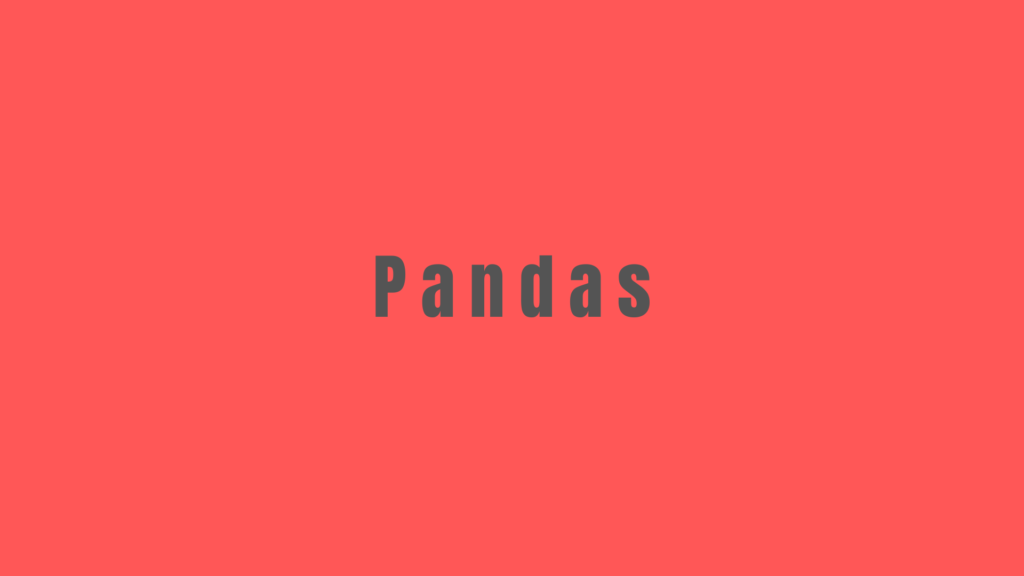
Essential Pandas Tips: 10 Code Snippets for Developers
Working with data in Python often means using the Pandas library, a powerful tool for data manipulation and analysis. However, the depth of Pandas can sometimes feel overwhelming. To help you work more efficiently, we’ve compiled 10 indispensable code snippets every Pandas developer should have in their toolkit. These snippets cover common tasks and clever tricks to boost your productivity.
1. Read Large Files in Chunks
Dealing with massive datasets? Instead of loading the entire file at once, use read_csv()
with chunks:
import pandas as pd
chunk_size = 100000
chunks = pd.read_csv('large_file.csv', chunksize=chunk_size)
for chunk in chunks:
process(chunk) # Replace with your processing logic
This approach helps you work with large files without exhausting memory.
2. Check for Missing Data
Missing values can wreak havoc on your analysis. Quickly identify missing data with this snippet:
import pandas as pd
missing_summary = df.isnull().sum()
print(missing_summary)
This prints a summary of missing values in each column, helping you take corrective actions.
3. Apply Functions to Columns
Transform data in a column efficiently with the apply()
method:
import pandas as pd
def clean_column(value):
return value.strip().lower()
df['cleaned_column'] = df['raw_column'].apply(clean_column)
This snippet standardizes column values, making them easier to analyze.
4. Filter Rows Based on Conditions
Filtering rows is a common task. Use this snippet to filter data based on multiple conditions:
filtered_df = df[(df['column1'] > 10) & (df['column2'] == 'value')]
This creates a new DataFrame containing only the rows that meet your criteria.
5. Group Data and Calculate Aggregates
Summarize your data by grouping it and calculating aggregates:
summary = df.groupby('category_column')['value_column'].sum()
print(summary)
Replace sum()
with other aggregation functions like mean()
, max()
, or count()
to suit your needs.
6. Sort Data by Multiple Columns
Organize your data by sorting it based on multiple columns:
sorted_df = df.sort_values(by=['column1', 'column2'], ascending=[True, False])
This sorts column1
in ascending order and column2
in descending order.
7. Add Calculated Columns
Create new columns based on existing ones without modifying the original data:
df['new_column'] = df['column1'] + df['column2']
This is handy for adding calculated metrics or combining columns.
8. Pivot Tables for Quick Insights
Generate a pivot table to analyze data across multiple dimensions:
pivot_table = pd.pivot_table(df, values='value_column', index='index_column', columns='category_column', aggfunc='sum')
print(pivot_table)
This snippet simplifies complex data exploration and summary tasks.
9. Save DataFrame to Multiple Formats
Export your DataFrame to various formats with ease:
df.to_csv('output.csv', index=False)
df.to_excel('output.xlsx', index=False)
df.to_json('output.json', orient='records')
This allows you to share your data in the format best suited for your audience.
10. Visualize Data with Pandas
While libraries like Matplotlib or Seaborn are popular for visualization, Pandas also provides built-in plotting capabilities:
df['column'].plot(kind='line')
plt.show()
For quick insights, use this built-in method to generate line, bar, or histogram plots.
Final Thoughts
These 10 snippets are designed to address common challenges faced by Pandas developers. By incorporating these into your workflow, you’ll be able to tackle data manipulation and analysis tasks more efficiently. Save these snippets, tweak them for your needs, and watch your productivity soar!