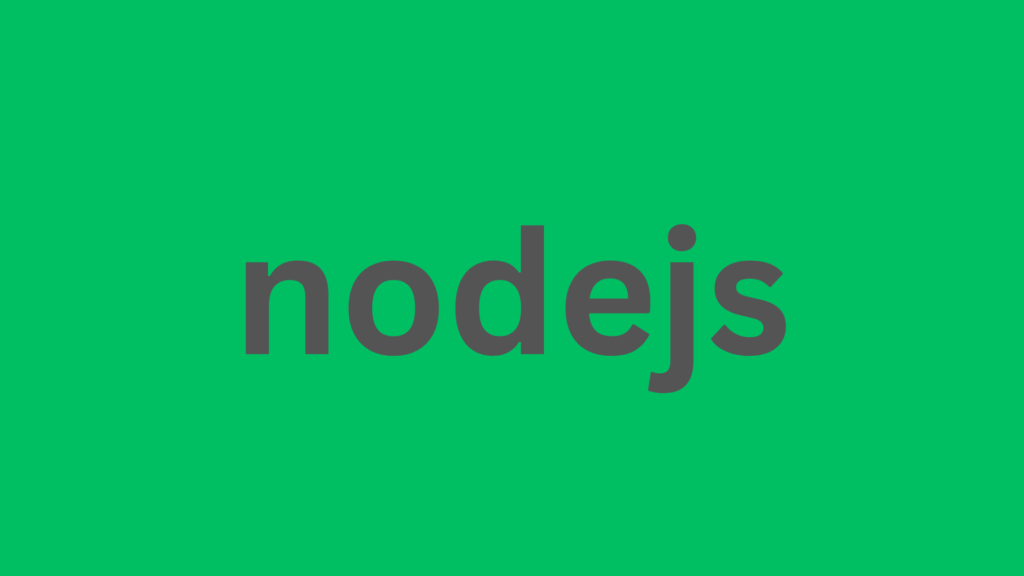
Think You Know Node.js? These 10 Quiz Questions Will Test Your Skills!
Node.js is a powerful tool for building fast, scalable network applications. As its popularity continues to soar, so does the need for developers to deepen their understanding of this runtime environment. Whether you’re a Node.js enthusiast or a seasoned developer, this quiz is designed to test your knowledge and give you a sense of how well you’ve mastered the essentials.
Below, you’ll find 10 thought-provoking questions covering key Node.js concepts. Grab a pen, a notebook (or just your brain), and let’s dive in!
Question 1: What is Node.js, and how does it differ from JavaScript in the browser?
Node.js is a runtime environment that allows developers to execute JavaScript outside the browser. Unlike JavaScript in the browser, which is mostly used for client-side interactions, Node.js enables server-side scripting. This means you can build scalable backend applications using the same language you use on the frontend.
Why it matters: Understanding this distinction helps developers write code optimized for server-side execution.
Question 2: What is the role of the V8 engine in Node.js?
The V8 engine, developed by Google, powers Node.js by executing JavaScript code. This engine compiles JavaScript into machine code, which significantly boosts performance.
Pro Tip: The V8 engine is the same one used in the Chrome browser, making it a critical component of Node.js’s speed and efficiency.
Question 3: Explain the Event Loop in Node.js.
The Event Loop is at the core of Node.js’s non-blocking, asynchronous behavior. It enables Node.js to handle multiple requests without creating a new thread for each one. Here’s a simplified breakdown of how it works:
- Incoming requests are added to the event queue.
- The event loop picks requests one by one and processes them.
- For tasks requiring I/O operations, the loop delegates them to worker threads, preventing delays.
Why it’s important: Mastering the Event Loop ensures you can build high-performance, non-blocking applications.
Question 4: What are Node.js modules, and how do you use them?
Node.js modules are reusable blocks of code that can be imported and exported within your application. Common module types include:
- Built-in modules (e.g.,
fs
,http
). - Custom modules (your own code).
- Third-party modules (via npm, like
express
).
Example:
javascriptCopyEditconst http = require('http');
http.createServer((req, res) => {
res.write('Hello, World!');
res.end();
}).listen(3000);
Question 5: How do you handle asynchronous operations in Node.js?
Node.js offers several methods for handling asynchronous tasks:
- Callbacks: The traditional way, but can lead to “callback hell.”
- Promises: Provide cleaner syntax for chaining operations.
- Async/Await: A modern approach for writing asynchronous code in a synchronous style.
Example with Async/Await:
javascriptCopyEditconst fs = require('fs').promises;
async function readFile() {
try {
const data = await fs.readFile('example.txt', 'utf8');
console.log(data);
} catch (err) {
console.error(err);
}
}
readFile();
Question 6: What is npm
, and why is it crucial for Node.js development?
npm
(Node Package Manager) is the default package manager for Node.js. It helps developers install, update, and manage dependencies for their projects. With millions of packages available, npm
is an invaluable tool for boosting productivity.
Quick Tip: Always use npm init
to create a package.json
file for managing your project dependencies.
Question 7: How do you manage environment variables in Node.js?
Environment variables store sensitive or environment-specific information, like API keys and database URLs. The dotenv
package is a popular tool for managing these variables securely.
Example:
- Install the package:
npm install dotenv
. - Create a
.env
file:makefileCopyEditAPI_KEY=your-api-key DB_URL=your-database-url
- Load variables in your app:javascriptCopyEdit
require('dotenv').config(); console.log(process.env.API_KEY);
Question 8: What are Streams in Node.js, and how are they used?
Streams are objects used to handle reading or writing data in chunks, making them ideal for processing large files. There are four types of streams in Node.js:
- Readable
- Writable
- Duplex
- Transform
Example: Reading a file using streams
javascriptCopyEditconst fs = require('fs');
const readStream = fs.createReadStream('largeFile.txt', 'utf8');
readStream.on('data', (chunk) => {
console.log(chunk);
});
Question 9: What is middleware in Node.js, and how is it used in frameworks like Express?
Middleware functions are executed sequentially during a request-response cycle in Node.js frameworks like Express. They can:
- Modify the request/response objects.
- Execute code.
- End the request-response cycle.
- Call the next middleware function.
Example in Express:
javascriptCopyEditconst express = require('express');
const app = express();
app.use((req, res, next) => {
console.log('Middleware executed');
next();
});
app.get('/', (req, res) => {
res.send('Hello, Middleware!');
});
app.listen(3000);
Question 10: How do you debug Node.js applications?
Debugging Node.js applications can be done using:
- The built-in
node inspect
command. - IDE features (like VS Code’s debugging tools).
- Third-party tools like
nodemon
ordebug
.
Quick Start with node inspect
:
bashCopyEditnode inspect app.js
Open Chrome DevTools to set breakpoints and step through your code.
Wrapping It Up
These 10 essential questions cover foundational topics every Node.js developer should know. By mastering these concepts, you’ll not only excel in coding but also in debugging, optimizing, and scaling Node.js applications. How did you do on the quiz? If any of the questions tripped you up, it’s time to revisit those topics and strengthen your understanding.
So, are you ready to tackle your next Node.js project like a pro? Let us know how you scored and what you’d like to learn next!