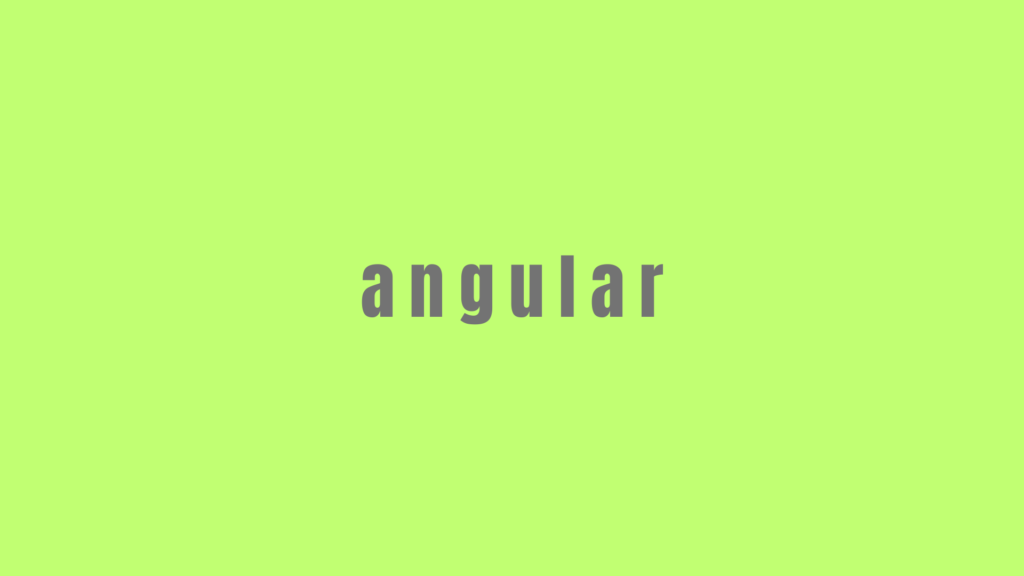
Think You Know AngularJS? Test Yourself with These Essential Questions!
Are you ready to test your Angular skills? Angular, as one of the most popular frameworks for building web applications, has evolved significantly over the years. Whether you’re a professional developer or just starting out, it’s essential to stay updated on its key concepts. This quiz challenges your knowledge of modern Angular, from components to dependency injection and state management. Let’s get started!
The Quiz
Here are 10 essential questions to test your Angular knowledge. Each question includes an in-depth answer and explanation, designed to help you solidify your understanding.
1. What is the purpose of Angular’s component-based architecture?
Angular’s component-based architecture is a core feature. Why is this approach beneficial for building web applications? Explain its advantages in terms of reusability and modularity.
Answer: Angular’s component-based architecture enables developers to build applications as a collection of small, reusable, and self-contained components. This makes it easier to manage and scale applications, promotes code reusability, and improves maintainability by isolating functionality.
2. How does Angular’s dependency injection system work, and why is it important?
Describe the role of Angular’s dependency injection (DI) system in application development. Provide an example to illustrate how DI improves code modularity and testing.
Answer: Dependency Injection in Angular provides a way to supply components or services with their dependencies rather than instantiating them directly. This allows better separation of concerns, easier testing, and reusability. For example, services can be injected into components using the constructor:
constructor(private myService: MyService) { }
This ensures that the component doesn’t create or manage service instances directly.
3. What are Angular’s lifecycle hooks, and how can you use them effectively?
Explain the purpose of Angular’s lifecycle hooks. Choose one hook and describe a use case for it.
Answer: Lifecycle hooks in Angular allow developers to tap into the different stages of a component’s lifecycle, such as initialization, rendering, and destruction. For example, the ngOnInit
hook is commonly used to fetch data or initialize properties when the component is initialized:
ngOnInit() {
this.loadData();
}
4. How does Angular handle reactive forms and template-driven forms?
What are the differences between these two approaches to building forms in Angular? When would you choose one over the other?
Answer: Reactive forms offer more control and are ideal for complex forms or dynamic validation. Template-driven forms are simpler and more declarative, making them suitable for basic forms. Reactive forms use the FormBuilder
and FormGroup
classes, while template-driven forms rely on Angular’s directives like ngModel
.
5. Explain the role of services in Angular and how they interact with components.
Why are services important in Angular applications? Provide an example of how services can be used to share data between components.
Answer: Services in Angular are singleton objects that encapsulate logic and data. They enable sharing of functionality and data across components. For instance, a data service can fetch API data and share it between multiple components:
@Injectable({ providedIn: 'root' })
export class DataService {
private data = new BehaviorSubject<string>('default');
currentData = this.data.asObservable();
changeData(newData: string) {
this.data.next(newData);
}
}
6. How does Angular’s Router enable navigation and lazy loading?
Describe how Angular’s Router is used to configure routes and implement lazy loading to improve performance.
Answer: The Angular Router enables navigation between different views or components. Lazy loading allows modules to load only when needed, reducing initial load time. For example:
const routes: Routes = [
{ path: 'home', loadChildren: () => import('./home/home.module').then(m => m.HomeModule) },
];
7. What is Angular’s Change Detection mechanism? How can you optimize it?
Discuss how Angular detects and updates changes in the DOM. Provide tips for optimizing performance in complex applications.
Answer: Angular’s Change Detection mechanism updates the DOM whenever the application state changes. For optimization, developers can use OnPush
change detection strategy and avoid unnecessary bindings:
@Component({
changeDetection: ChangeDetectionStrategy.OnPush
})
export class MyComponent {
}
8. How do Angular modules contribute to application structure?
Explain the role of NgModules in organizing Angular applications. How do feature modules improve scalability?
Answer: NgModules group related components, directives, pipes, and services into cohesive units. Feature modules allow developers to break the application into manageable parts, improving scalability and code organization.
9. What are RxJS Observables, and how are they used in Angular?
Explain the role of RxJS in Angular. Provide an example of using Observables for asynchronous data streams.
Answer: RxJS Observables are used in Angular to handle asynchronous data streams, such as API calls or user events. For example:
this.httpClient.get('api/data').subscribe(data => {
console.log(data);
});
10. How does Angular’s state management with NgRx work?
Describe the concept of state management and how NgRx simplifies it. Provide a brief overview of its key components.
Answer: NgRx provides a reactive state management solution using Redux patterns. Key components include Actions, Reducers, and Stores. Actions describe state changes, Reducers handle state updates, and Stores hold the application’s state:
store.dispatch(new UpdateDataAction(newData));
store.select('data').subscribe(data => console.log(data));
Why This Quiz Matters
Angular’s latest versions introduce powerful features that simplify complex development tasks. Mastering these concepts is crucial for staying relevant and building scalable, efficient web applications. Whether you’re preparing for a job interview or aiming to improve your skills, these questions will deepen your understanding of Angular’s core principles.
So, how many questions did you answer confidently? Share your thoughts and feedback in the comments below!