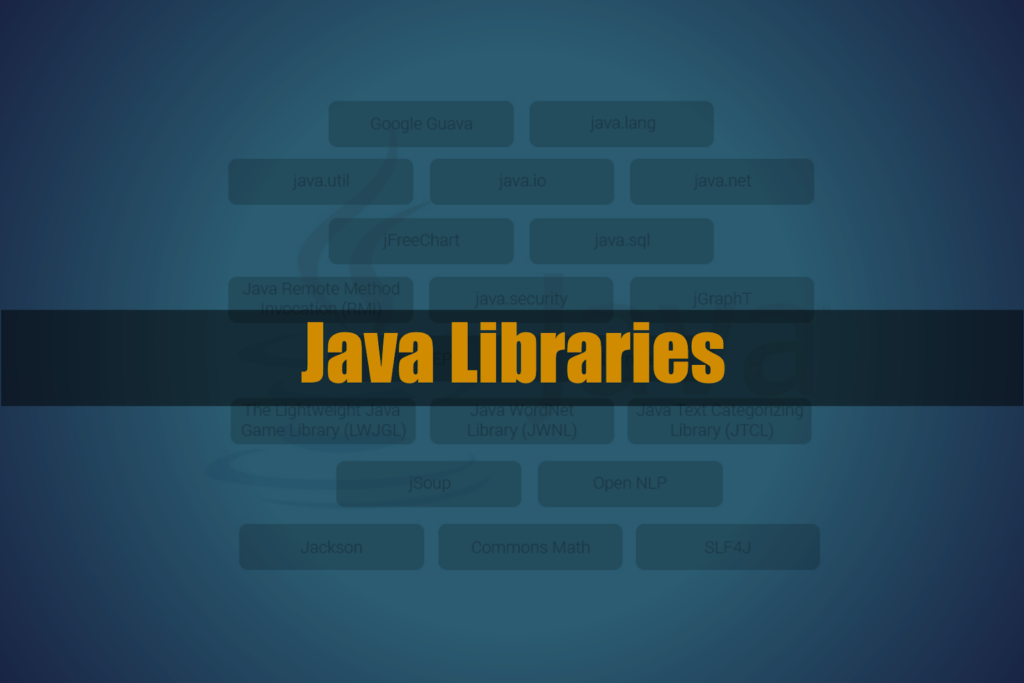
Best Open Source Java Libraries and Core Utilities: Complete Guide
Java’s ecosystem is rich with powerful open-source libraries that can significantly enhance your development experience and application performance. In this comprehensive guide, we’ll explore the most essential Java utilities and core libraries that every developer should know about. From simplifying collections handling to eliminating boilerplate code, these libraries will transform the way you write Java applications.
Apache Commons: The Swiss Army Knife of Java Libraries
Apache Commons stands as one of the most mature and comprehensive collections of open-source utilities for Java developers. This project consists of several components, each addressing specific development needs:
Commons Lang
The Commons Lang library provides a wealth of helper utilities for Java’s core classes, particularly for working with strings, numbers, and Object manipulation. Consider these practical examples:
javaCopyimport org.apache.commons.lang3.StringUtils;
// String manipulation made easy
String input = null;
boolean isEmpty = StringUtils.isEmpty(input); // true
boolean isBlank = StringUtils.isBlank(" "); // true
String reversed = StringUtils.reverse("Hello"); // "olleH"
// Object utilities
import org.apache.commons.lang3.ObjectUtils;
String result = ObjectUtils.defaultIfNull(nullableString, "default");
Commons Collections
This component extends Java’s collection framework with additional data structures and utilities:
javaCopyimport org.apache.commons.collections4.CollectionUtils;
// Powerful collection operations
List<String> list1 = Arrays.asList("A", "B", "C");
List<String> list2 = Arrays.asList("B", "C", "D");
Collection<String> union = CollectionUtils.union(list1, list2);
Collection<String> intersection = CollectionUtils.intersection(list1, list2);
Guava: Google’s Gift to Java Developers
Google’s Guava library offers a rich set of utilities that complement Java’s standard libraries. It’s designed with performance and clean code in mind:
Immutable Collections
javaCopyimport com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
ImmutableList<String> immutableList = ImmutableList.of("one", "two", "three");
ImmutableMap<String, Integer> immutableMap = ImmutableMap.of(
"one", 1,
"two", 2,
"three", 3
);
Powerful String Utilities
javaCopyimport com.google.common.base.Joiner;
import com.google.common.base.Splitter;
// String joining with null handling
String joined = Joiner.on(",")
.skipNulls()
.join("foo", null, "bar"); // Returns "foo,bar"
// Smart string splitting
Iterable<String> pieces = Splitter.on(',')
.trimResults()
.omitEmptyStrings()
.split("foo, bar,, baz");
Project Lombok: The Boilerplate Killer
Lombok has revolutionized Java development by eliminating countless lines of repetitive code through annotations:
javaCopyimport lombok.Data;
import lombok.Builder;
@Data
@Builder
public class User {
private String username;
private String email;
private int age;
private boolean active;
}
This simple annotation generates:
- Getters and setters
- toString() method
- equals() and hashCode()
- A builder pattern implementation
Eclipse Collections: High-Performance Collections Framework
Eclipse Collections provides rich, high-performance collection APIs that go beyond Java’s standard collections:
javaCopyimport org.eclipse.collections.api.list.MutableList;
import org.eclipse.collections.impl.list.mutable.FastList;
MutableList<String> list = FastList.newList();
list.with("One", "Two", "Three")
.select(s -> s.length() > 3)
.collect(String::toUpperCase);
Time Handling: JodaTime and Java Time
While Java 8+ includes the excellent java.time package, some projects still use JodaTime, and understanding both is valuable:
Java Time (Java 8+)
javaCopyimport java.time.LocalDateTime;
import java.time.ZonedDateTime;
import java.time.format.DateTimeFormatter;
LocalDateTime now = LocalDateTime.now();
ZonedDateTime zonedDateTime = ZonedDateTime.now();
String formatted = now.format(DateTimeFormatter.ISO_LOCAL_DATE_TIME);
JodaTime (for legacy projects)
javaCopyimport org.joda.time.DateTime;
import org.joda.time.format.DateTimeFormat;
DateTime dateTime = new DateTime();
String formatted = dateTime.toString(DateTimeFormat.forPattern("yyyy-MM-dd HH:mm"));
Additional Essential Libraries
SLF4J with Logback
The de facto standard for Java logging:
javaCopyimport org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyClass {
private static final Logger logger = LoggerFactory.getLogger(MyClass.class);
public void doSomething() {
logger.info("Operation started");
try {
// operation code
} catch (Exception e) {
logger.error("Operation failed", e);
}
}
}
AssertJ
Modern fluent assertions for Java:
javaCopyimport static org.assertj.core.api.Assertions.*;
@Test
public void testAssertions() {
List<String> list = Arrays.asList("Java", "Python", "JavaScript");
assertThat(list)
.hasSize(3)
.contains("Java")
.doesNotContain("Ruby");
}
Integration Tips
When using multiple libraries together, consider these best practices:
- Dependency Management: Use Maven or Gradle to manage library versions and resolve conflicts.
- Version Compatibility: Ensure all libraries are compatible with your Java version.
- Performance Monitoring: Monitor memory usage when using collection libraries extensively.
- Documentation: Keep track of which libraries are used for what purpose in your project documentation.
Conclusion
These open-source Java libraries and utilities can significantly improve your development efficiency and code quality. While it’s not necessary to use all of them in every project, understanding their capabilities helps you choose the right tools for your specific needs.