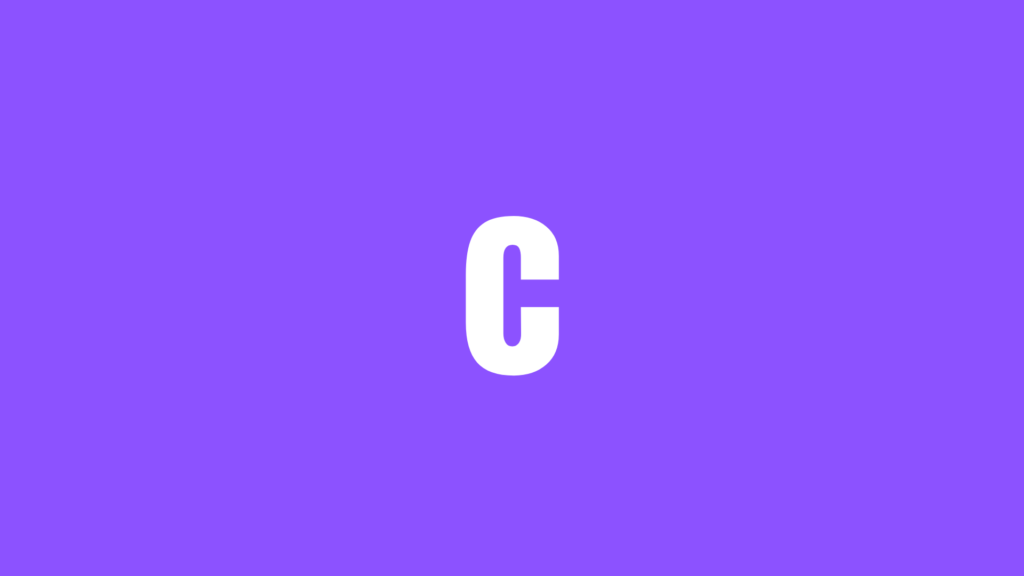
C Programming: String Copy Functions Compared – strcpy, strncpy, and strlcpy Tutorial
Buffer overflows have long been one of the most notorious security vulnerabilities in C programming. At the heart of many such issues lies string manipulation, particularly string copying operations. Understanding the differences between strcpy, strncpy, and strlcpy isn’t just about writing better code – it’s about writing secure code.
The Evolution of String Copying in C
When C was first developed, string manipulation seemed straightforward. The standard library provided strcpy(), a simple function to copy strings. However, as security concerns grew and buffer overflows became a significant threat, newer alternatives emerged: strncpy() and later, strlcpy(). Each function has its own strengths and pitfalls, making the choice between them crucial for developers.
Understanding strcpy()
The simplest and oldest of the three functions, strcpy() copies a string from source to destination, including the null terminator. Its prototype looks like this:
cCopychar *strcpy(char *dest, const char *src);
Advantages of strcpy()
- Simple to use and understand
- Guaranteed null termination
- Efficient performance
- Part of the standard C library
Disadvantages of strcpy()
- No buffer overflow protection
- Requires pre-validated input
- Can cause security vulnerabilities
- Unsafe for untrusted data
Consider this example:
cCopychar small_buffer[5];
char *long_string = "This string is too long";
strcpy(small_buffer, long_string); // Buffer overflow!
This code will write beyond small_buffer’s bounds, potentially corrupting memory or causing a crash.
Enter strncpy()
To address strcpy()’s security issues, strncpy() was introduced. It allows specifying a maximum number of characters to copy:
cCopychar *strncpy(char *dest, const char *src, size_t n);
Advantages of strncpy()
- Prevents buffer overflows
- Part of the standard C library
- Allows precise control over copy length
- Suitable for fixed-width fields
Disadvantages of strncpy()
- Doesn’t guarantee null termination
- May leave destination unterminated
- Inefficient for large buffers
- Fills remaining space with null bytes
Here’s a proper usage example:
cCopychar dest[10];
strncpy(dest, "Hello", 9);
dest[9] = '\0'; // Explicit null termination
The Modern Solution: strlcpy()
Developed for OpenBSD, strlcpy() aims to combine safety with ease of use:
cCopysize_t strlcpy(char *dest, const char *src, size_t size);
Advantages of strlcpy()
- Always null terminates
- Returns intended string length
- More efficient than strncpy()
- Clearer semantics
Disadvantages of strlcpy()
- Not part of standard C library
- Limited platform availability
- Requires external implementation
- May need portability wrapper
Example usage:
cCopychar dest[10];
size_t result = strlcpy(dest, "Hello, World!", sizeof(dest));
if (result >= sizeof(dest)) {
// String was truncated
}
Performance Considerations
When it comes to performance, these functions show interesting characteristics:
- strcpy() is fastest but unsafe
- strncpy() can be slower due to null padding
- strlcpy() offers good performance without padding
Best Practices for String Copying
When to Use Each Function
Use strcpy() when:
- Source string length is known
- Destination buffer size is verified
- Performance is critical
- Working with trusted data
Use strncpy() when:
- Working with fixed-width fields
- Null padding is desired
- Standard C compliance is required
- Buffer size is known
Use strlcpy() when:
- Security is paramount
- Platform supports it
- Clear error handling is needed
- Working with unknown data
Security Guidelines
- Always validate input lengths
- Check function return values
- Ensure proper buffer sizing
- Consider using string libraries
Modern Alternatives
While these traditional functions remain widely used, modern C programming often employs alternatives:
- String Classes
- Safe String Libraries
- Buffer Management Systems
- String View Types
Common Pitfalls to Avoid
strcpy() Pitfalls
- Assuming source fits destination
- Not checking for NULL pointers
- Overlapping memory regions
- Trusting user input
strncpy() Pitfalls
- Forgetting manual null termination
- Incorrect size calculations
- Assuming automatic null termination
- Inefficient null padding
strlcpy() Pitfalls
- Platform dependency issues
- Ignoring return values
- Assuming universal availability
- Incorrect size parameter
Conclusion
Choosing between strcpy(), strncpy(), and strlcpy() involves balancing security, performance, and portability. While strcpy() offers simplicity and speed, its security risks make it suitable only for carefully controlled scenarios. strncpy() provides better safety but requires careful handling of null termination. strlcpy() represents the most modern approach, combining safety and usability, though its limited availability can be a constraint.
For modern C programming, strlcpy() is often the best choice when available. Otherwise, carefully used strncpy() with explicit null termination provides a good balance of safety and portability. Remember: in string manipulation, the extra effort spent on safety always pays off in the long run.