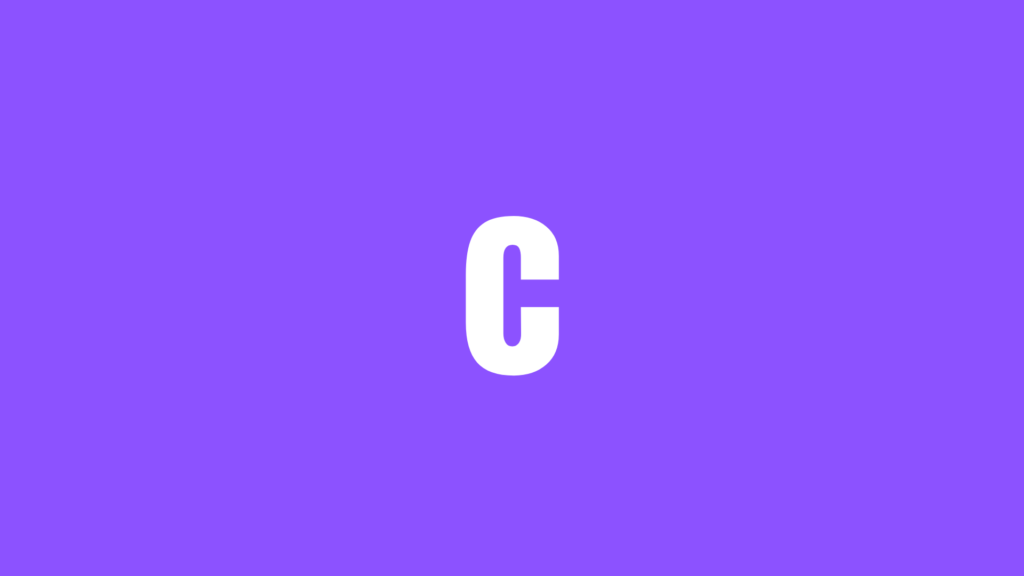
C String Manipulation Decoded: Choosing the Right Copy Function
When it comes to dynamic memory allocation in programming, two key players often take center stage: malloc
and calloc
. These C standard library functions are vital tools for developers who need to allocate memory at runtime. But despite being cut from the same cloth, malloc
and calloc
differ in functionality, use cases, and performance.
In this article, we’ll unravel the mystery behind these memory management twins, explore their differences, and provide practical examples to help you master their usage.
What Is Dynamic Memory Allocation?
Dynamic memory allocation is a way for programs to request memory from the operating system during runtime. Unlike static memory allocation, where memory size is predetermined at compile time, dynamic allocation offers flexibility by allowing memory to be allocated as needed.
This is particularly useful when dealing with data structures like arrays, linked lists, or trees whose sizes may not be known in advance.
Enter malloc
(short for memory allocation) and calloc
(short for contiguous allocation), two functions that allow you to dynamically allocate memory. Let’s dive into their specifics.
What Is malloc
?
malloc
stands for memory allocation. It’s a function that allocates a block of memory of a specified size and returns a pointer to the beginning of the block.
Syntax
cCopyEditvoid* malloc(size_t size);
- size_t size: The number of bytes to allocate.
- Return Value: A pointer to the allocated memory, or
NULL
if the allocation fails.
Key Characteristics of malloc
- Uninitialized Memory: The memory allocated by
malloc
is not initialized, meaning it may contain garbage values. - Flexibility: You can allocate a custom size of memory, depending on your needs.
Example Usage
cCopyEdit#include <stdio.h>
#include <stdlib.h>
int main() {
int* ptr = (int*) malloc(5 * sizeof(int)); // Allocates memory for 5 integers
if (ptr == NULL) {
printf("Memory allocation failed.\n");
return 1;
}
for (int i = 0; i < 5; i++) {
ptr[i] = i + 1; // Assigning values
printf("%d ", ptr[i]);
}
free(ptr); // Freeing allocated memory
return 0;
}
What Is calloc
?
calloc
stands for contiguous allocation. It’s designed to allocate memory for an array of elements, initializing all memory locations to zero.
Syntax
cCopyEditvoid* calloc(size_t num, size_t size);
- size_t num: The number of elements to allocate.
- size_t size: The size of each element in bytes.
- Return Value: A pointer to the allocated memory, or
NULL
if the allocation fails.
Key Characteristics of calloc
- Zero Initialization: Unlike
malloc
,calloc
initializes all allocated memory to zero. - Contiguous Blocks: It ensures the memory allocated is contiguous and suited for arrays.
Example Usage
cCopyEdit#include <stdio.h>
#include <stdlib.h>
int main() {
int* ptr = (int*) calloc(5, sizeof(int)); // Allocates and initializes memory for 5 integers
if (ptr == NULL) {
printf("Memory allocation failed.\n");
return 1;
}
for (int i = 0; i < 5; i++) {
printf("%d ", ptr[i]); // Prints 0 as memory is initialized
}
free(ptr); // Freeing allocated memory
return 0;
}
Key Differences Between malloc
and calloc
Feature | malloc | calloc |
---|---|---|
Initialization | Memory is uninitialized | Memory is initialized to zero |
Parameters | One parameter: size in bytes | Two parameters: number of elements and size of each element |
Use Case | General-purpose allocation | Allocating arrays or zero-initialized memory |
Performance | Slightly faster (no initialization overhead) | Slower due to zero initialization |
When to Use malloc
or calloc
?
- Use
malloc
when initialization isn’t a priority and you want faster allocation. For example, allocating memory for temporary buffers. - Use
calloc
when zero-initialization is critical, such as when creating arrays where all elements should initially be zero.
Common Pitfalls and Best Practices
1. Forgetting to Free Memory
Memory allocated with malloc
or calloc
is not automatically deallocated. Use free()
to avoid memory leaks.
cCopyEditfree(ptr);
2. Not Checking for NULL
Always check if the memory allocation was successful.
cCopyEditif (ptr == NULL) {
printf("Memory allocation failed.\n");
}
3. Overusing calloc
While calloc
ensures zero initialization, its overhead may not be necessary for all use cases. Consider your requirements before choosing.
4. Casting the Return Value
In C, casting the return value of malloc
or calloc
is unnecessary. However, it’s required in C++ for type safety.
Performance Considerations
Since malloc
skips initialization, it is generally faster than calloc
. However, the difference is negligible for small allocations. The choice between the two should depend on the context and specific needs of your program.
Conclusion
Understanding the differences between malloc
and calloc
is crucial for efficient memory management in C and C++ programming. While both are powerful tools for dynamic memory allocation, their unique characteristics make them suitable for different scenarios.
Whether you’re writing a small utility or a complex application, knowing when to use malloc
or calloc
will make your code cleaner, more efficient, and less prone to errors. So, the next time you’re faced with a memory allocation challenge, you’ll know exactly which tool to reach for!