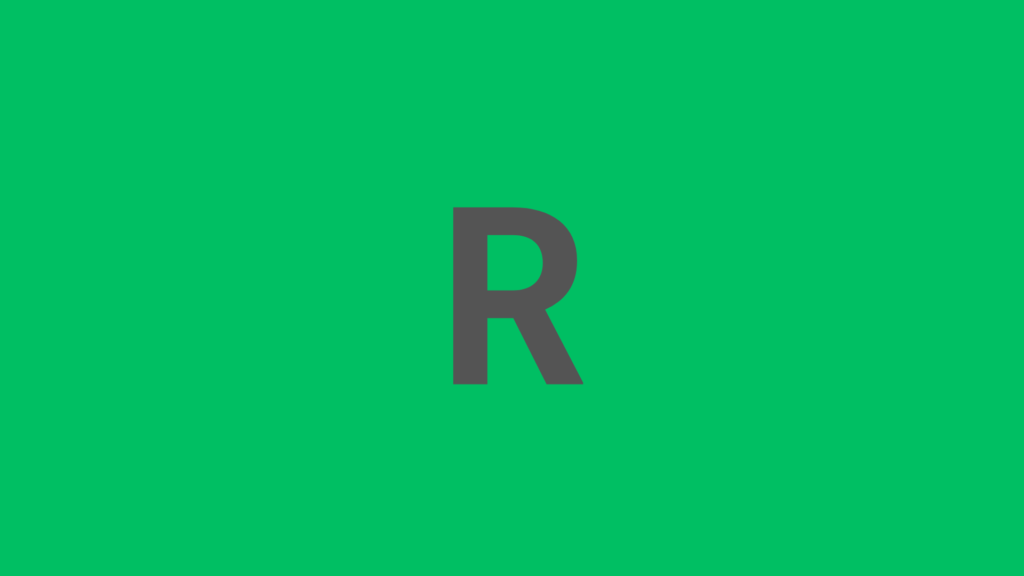
R Programming Tutorial: Step-by-Step Guide with Practical Examples
In today’s data-driven world, R programming has emerged as a powerhouse for data analysis, statistical computing, and visualization. Whether you’re a budding data scientist, researcher, or analyst, learning R can open doors to endless possibilities in data manipulation and analysis. This comprehensive guide will walk you through everything you need to know about getting started with R programming.
Getting Started with R Programming
Installing R and RStudio
Your journey begins with setting up your development environment. First, download R from the Comprehensive R Archive Network (CRAN). After installing R, download RStudio, an integrated development environment (IDE) that makes R programming more intuitive and efficient.
rCopy# Your first R command
print("Hello, World!")
Understanding R’s Basic Syntax
R’s syntax is designed to be intuitive for statistical computing. Here are the fundamental concepts:
- Variables and Data Types
rCopy# Numeric
age <- 25
# Character
name <- "John"
# Logical
is_student <- TRUE
- Data Structures R offers various data structures:
- Vectors:
numbers <- c(1, 2, 3, 4, 5)
- Lists:
my_list <- list(name="John", age=25)
- Data Frames:
data <- data.frame(name=c("John","Jane"), age=c(25,30))
- Matrices:
matrix(1:9, nrow=3, ncol=3)
Essential R Programming Concepts
Working with Data
Data manipulation is R’s strength. The dplyr
package makes data wrangling straightforward:
rCopy# Install and load dplyr
install.packages("dplyr")
library(dplyr)
# Example of data manipulation
mtcars %>%
filter(mpg > 20) %>%
select(mpg, cyl, wt) %>%
arrange(desc(mpg))
Data Visualization
R’s visualization capabilities are exceptional, particularly with ggplot2
:
rCopy# Install and load ggplot2
install.packages("ggplot2")
library(ggplot2)
# Create a basic plot
ggplot(mtcars, aes(x=wt, y=mpg)) +
geom_point() +
geom_smooth(method="lm") +
labs(title="Car Weight vs. MPG",
x="Weight (1000 lbs)",
y="Miles per Gallon")
Advanced R Programming Techniques
Statistical Analysis
R excels in statistical computing:
rCopy# Perform linear regression
model <- lm(mpg ~ wt + hp, data=mtcars)
summary(model)
# Conduct t-test
t.test(mtcars$mpg ~ mtcars$am)
Writing Functions
Custom functions enhance code reusability:
rCopycalculate_bmi <- function(weight, height) {
bmi <- weight / (height^2)
return(bmi)
}
# Use the function
my_bmi <- calculate_bmi(70, 1.75)
Best Practices for R Programming
Code Organization
- Use clear, descriptive variable names
- Comment your code thoroughly
- Break complex operations into smaller functions
- Use consistent formatting
Performance Optimization
- Vectorize operations when possible
- Use appropriate data structures
- Avoid growing objects in loops
- Utilize parallel processing for large datasets
Popular R Packages for Different Applications
Data Science Packages
tidyverse
: Collection of data science toolscaret
: Machine learning workflowsshiny
: Interactive web applicationsplotly
: Interactive visualizations
Statistical Packages
stats
: Built-in statistical functionslme4
: Mixed-effects modelssurvival
: Survival analysisforecast
: Time series analysis
Real-World Applications
Data Analysis Example
rCopy# Load and analyze dataset
library(tidyverse)
data(diamonds)
diamonds %>%
group_by(cut) %>%
summarize(
avg_price = mean(price),
count = n()
) %>%
arrange(desc(avg_price))
Creating Reports with R Markdown
R Markdown enables creating professional reports:
rCopy---
title: "Data Analysis Report"
author: "Your Name"
date: "2025-01-19"
output: html_document
---
Getting Help and Resources
- Online Resources
- R Documentation
- Stack Overflow
- R-bloggers
- DataCamp tutorials
- Community Support
- R User Groups
- GitHub repositories
- R-Ladies communities
- Stack Exchange
Conclusion
R programming is a valuable skill that can significantly enhance your data analysis capabilities. By following this guide and practicing regularly, you’ll be well on your way to mastering R. Remember, the key to success is consistent practice and exploration of R’s vast ecosystem of packages and tools.
Start with basic concepts, gradually move to more complex operations, and don’t hesitate to experiment with different packages and functions. The R community is welcoming and supportive, making it easier to find help when needed.
Whether you’re analyzing data, creating visualizations, or building statistical models, R provides the tools you need to succeed in your data science journey. Keep learning, keep coding, and most importantly, enjoy the process of discovering what R can do for you.