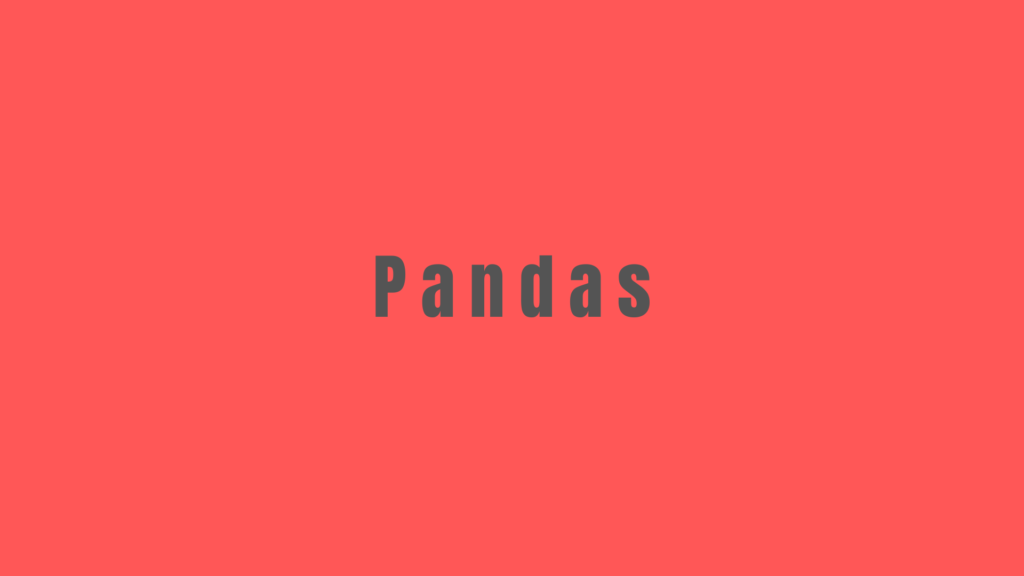
Say Goodbye to Unwanted Data: Mastering Row Deletion in Pandas
Data cleaning is an essential part of any data analysis process. Whether you’re working on a massive dataset or a small collection of records, cleaning your data ensures accurate results and efficient analysis. One common task in data cleaning is removing rows that are irrelevant, erroneous, or incomplete. If you’re using Python and the Pandas library, you’re in luck—it provides simple, powerful ways to handle this.
In this article, we’ll explore how to drop rows in Pandas step by step, with practical examples and useful tips.
Why Would You Drop Rows in Pandas?
Before diving into the technicalities, let’s first understand why you might want to drop rows from your dataset:
- Duplicate Data: Repeated rows can distort your analysis.
- Missing Values: Rows with incomplete data can be useless for certain operations.
- Irrelevant Records: Some data might not fit the criteria for your analysis.
- Error Correction: Mistakes in data entry can lead to faulty rows.
Now that we know the why, let’s move to the how.
The Basics of Pandas
Pandas is a popular Python library used for data manipulation and analysis. A core concept in Pandas is the DataFrame, which is essentially a table-like structure where data is stored in rows and columns.
To drop a row in Pandas, you’ll primarily use the .drop()
method. Let’s break it down with examples.
1. Dropping Rows by Index
If you know the specific index of the row you want to remove, the .drop()
method makes this straightforward.
Here’s an example:
pythonCopyEditimport pandas as pd
# Sample DataFrame
data = {'Name': ['Alice', 'Bob', 'Charlie', 'David'],
'Age': [25, 30, 35, 40]}
df = pd.DataFrame(data)
print("Original DataFrame:")
print(df)
# Dropping the row at index 1 (Bob)
df = df.drop(index=1)
print("\nDataFrame after dropping row with index 1:")
print(df)
Output:
markdownCopyEditOriginal DataFrame:
Name Age
0 Alice 25
1 Bob 30
2 Charlie 35
3 David 40
DataFrame after dropping row with index 1:
Name Age
0 Alice 25
2 Charlie 35
3 David 40
Here, the drop
method removed the row with the index 1
.
2. Dropping Rows Based on Conditions
Sometimes, you want to remove rows that meet specific criteria. For example, let’s say you want to drop all rows where the Age
is greater than 30:
pythonCopyEdit# Dropping rows where Age > 30
df = df[df['Age'] <= 30]
print("\nDataFrame after dropping rows where Age > 30:")
print(df)
Output:
sqlCopyEditDataFrame after dropping rows where Age > 30:
Name Age
0 Alice 25
1 Bob 30
This method filters the DataFrame by retaining only rows that satisfy the condition (Age <= 30
).
3. Dropping Duplicate Rows
Duplicate rows can often sneak into datasets. Pandas makes it simple to remove them with the drop_duplicates()
method:
pythonCopyEdit# Sample DataFrame with duplicates
data = {'Name': ['Alice', 'Bob', 'Alice', 'David'],
'Age': [25, 30, 25, 40]}
df = pd.DataFrame(data)
print("Original DataFrame with duplicates:")
print(df)
# Dropping duplicates
df = df.drop_duplicates()
print("\nDataFrame after dropping duplicates:")
print(df)
Output:
markdownCopyEditOriginal DataFrame with duplicates:
Name Age
0 Alice 25
1 Bob 30
2 Alice 25
3 David 40
DataFrame after dropping duplicates:
Name Age
0 Alice 25
1 Bob 30
3 David 40
The drop_duplicates
method removes repeated rows while keeping the first occurrence.
4. Dropping Rows with Missing Values
Datasets often contain missing or null values. You can easily remove these rows using the dropna()
method:
pythonCopyEdit# Sample DataFrame with missing values
data = {'Name': ['Alice', 'Bob', None, 'David'],
'Age': [25, 30, None, 40]}
df = pd.DataFrame(data)
print("Original DataFrame with missing values:")
print(df)
# Dropping rows with missing values
df = df.dropna()
print("\nDataFrame after dropping rows with missing values:")
print(df)
Output:
sqlCopyEditOriginal DataFrame with missing values:
Name Age
0 Alice 25.0
1 Bob 30.0
2 None NaN
3 David 40.0
DataFrame after dropping rows with missing values:
Name Age
0 Alice 25.0
1 Bob 30.0
3 David 40.0
5. Dropping Rows In-Place
By default, the .drop()
method creates a new DataFrame. If you want to modify the existing DataFrame directly, use the inplace=True
parameter:
pythonCopyEdit# Dropping a row in-place
df.drop(index=0, inplace=True)
print("\nDataFrame after dropping row with index 0 in-place:")
print(df)
Key Takeaways
- The
.drop()
method is versatile, allowing you to remove rows by index or labels. - Use conditional filtering to drop rows that meet specific criteria.
- Handle duplicates with
drop_duplicates()
and missing values withdropna()
. - Modify DataFrames directly with
inplace=True
if needed.
Whether you’re cleaning survey responses, preparing financial data, or working on machine learning datasets, mastering these techniques will make your data manipulation tasks seamless.
Now that you’ve learned how to drop rows in Pandas, you’re one step closer to becoming a data-cleaning wizard. Go ahead, clean that data, and let your analysis shine!