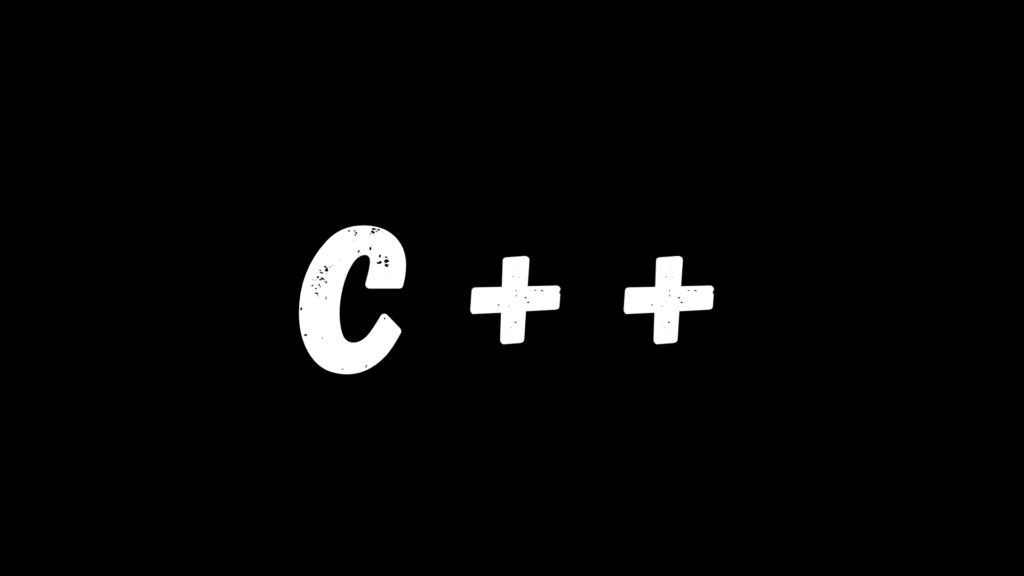
C++ Quiz Challenge: Test Your Programming Prowess!
Are you confident in your C++ programming skills? Whether you’re preparing for a technical interview or simply want to test your knowledge, this comprehensive quiz will challenge your understanding of essential C++ concepts. Let’s dive into ten carefully crafted questions that every developer should be able to answer.
1. Smart Pointer Fundamentals
Consider this code snippet:
cppCopystd::unique_ptr<int> ptr1(new int(42));
std::unique_ptr<int> ptr2 = ptr1;
What happens when you try to compile and execute this code? More importantly, why?
The above code will fail to compile. This exemplifies one of the fundamental principles of unique_ptr
: it cannot be copied, only moved. This restriction ensures single ownership semantics, preventing multiple pointers from managing the same resource. To transfer ownership, you would need to use std::move
:
cppCopystd::unique_ptr<int> ptr2 = std::move(ptr1);
2. The Virtual Destructor Puzzle
Let’s examine this inheritance scenario:
cppCopyclass Base {
public:
~Base() { }
};
class Derived : public Base {
public:
Derived() { resource = new int[1000]; }
~Derived() { delete[] resource; }
private:
int* resource;
};
int main() {
Base* ptr = new Derived();
delete ptr;
}
Is there a potential issue here? If so, what’s the solution?
This code contains a subtle but dangerous memory leak. When we delete through a base class pointer, if the destructor isn’t virtual, only the base class destructor is called. The solution is to declare the base class destructor as virtual:
cppCopyvirtual ~Base() { }
3. Template Metaprogramming Challenge
Can you spot what’s unique about this factorial implementation?
cppCopytemplate<unsigned int N>
struct Factorial {
static const unsigned int value = N * Factorial<N-1>::value;
};
template<>
struct Factorial<0> {
static const unsigned int value = 1;
};
This demonstrates compile-time computation using template metaprogramming. The factorial is calculated during compilation, not at runtime, resulting in zero runtime overhead. This technique is particularly useful for optimizing performance-critical code.
4. The const Conundrum
What’s the difference between these declarations?
cppCopyconst int* ptr1;
int const* ptr2;
int* const ptr3;
This question tests understanding of const placement:
const int*
andint const*
are equivalent: pointer to a constant integerint* const
is different: constant pointer to an integer Remember: read from right to left, const applies to what’s immediately to its left (or right if nothing’s to the left).
5. Rule of Five Implementation
Modern C++ emphasizes the “Rule of Five.” What five special member functions should you consider implementing?
The Rule of Five states that if you implement any of these, you typically need all five:
- Destructor
- Copy constructor
- Copy assignment operator
- Move constructor
- Move assignment operator
Here’s a practical example:
cppCopyclass ResourceManager {
public:
~ResourceManager(); // Destructor
ResourceManager(const ResourceManager&); // Copy constructor
ResourceManager& operator=(const ResourceManager&); // Copy assignment
ResourceManager(ResourceManager&&) noexcept; // Move constructor
ResourceManager& operator=(ResourceManager&&) noexcept; // Move assignment
};
6. Lambda Expression Mastery
What will this code output?
cppCopyint multiplier = 10;
auto lambda = [multiplier](int x) { return x * multiplier; };
multiplier = 20;
std::cout << lambda(5);
This outputs 50, not 100, because the lambda captures multiplier
by value at the time of creation. To capture changes to multiplier
, you’d need to capture by reference using [&multiplier]
.
7. RAII Pattern Implementation
Why is RAII (Resource Acquisition Is Initialization) important in C++? Consider this example:
cppCopyclass FileHandler {
std::fstream file;
public:
FileHandler(const std::string& filename) : file(filename) { }
~FileHandler() { if(file.is_open()) file.close(); }
};
RAII ensures resource management is tied to object lifetime, preventing resource leaks even when exceptions occur. This pattern is fundamental to C++ and is used extensively in the standard library.
8. STL Algorithm Challenge
How would you find the first element greater than 42 in a vector using STL algorithms?
cppCopystd::vector<int> numbers = {10, 20, 30, 40, 50, 60};
auto it = std::find_if(numbers.begin(), numbers.end(),
[](int n) { return n > 42; });
This demonstrates effective use of STL algorithms combined with lambda expressions, a powerful combination in modern C++.
9. Perfect Forwarding Understanding
What’s special about this function template?
cppCopytemplate<typename T>
void wrapper(T&& arg) {
function(std::forward<T>(arg));
}
This implements perfect forwarding, preserving the value category (lvalue/rvalue) of the original argument. The std::forward
ensures efficient handling of both lvalue and rvalue arguments.
10. Memory Order and Atomics
In concurrent programming, what’s the significance of this code?
cppCopystd::atomic<int> counter{0};
counter.store(1, std::memory_order_release);
int value = counter.load(std::memory_order_acquire);
This demonstrates understanding of memory ordering in concurrent programming. The release-acquire ordering ensures that all writes before the store are visible to reads after the load, crucial for thread synchronization.
Conclusion
How did you fare with these questions? Each one highlights important aspects of C++ programming that developers should understand. Regular practice with such concepts not only prepares you for technical interviews but also makes you a more effective C++ programmer.
Remember, C++ is a complex language with many nuances. Understanding these fundamental concepts helps you write more efficient, maintainable, and bug-free code. Keep practicing and expanding your knowledge!